Creating a blurred window background with SwiftUI on macOS
I’ve been experimenting with using SwiftUI for some native macOS apps, and had trouble figuring this out initially. If you want a modern blurred window background for your macOS app from SwiftUI, there’s not a built-in way to do it (or at least that I could find, if there is, let me know!). Luckily, the code to do it is short and simple.
First, we need to set the background of the view to a NSVisualEffectView
. That class or an equivalent is not yet available in SwiftUI, so we have to create a NSViewRepresentable
to expose it to SwiftUI:
struct VisualEffectView: NSViewRepresentable {
func makeNSView(context: Context) -> NSVisualEffectView {
let effectView = NSVisualEffectView()
effectView.state = .active
return effectView
}
func updateNSView(_ nsView: NSVisualEffectView, context: Context) {
}
}
Once you have that, you can add it as a background
to your window’s main content view. The ignoresSafeArea()
isn’t required, but looks better if you want to use the full-size content view and let the content go under the window titlebar.
struct BlurredWindowContentView: View {
var body: some View {
content
.background(VisualEffectView().ignoresSafeArea())
}
}
And that’s all you need to do. Here’s a screenshot of what that looks like as of macOS 12.3:
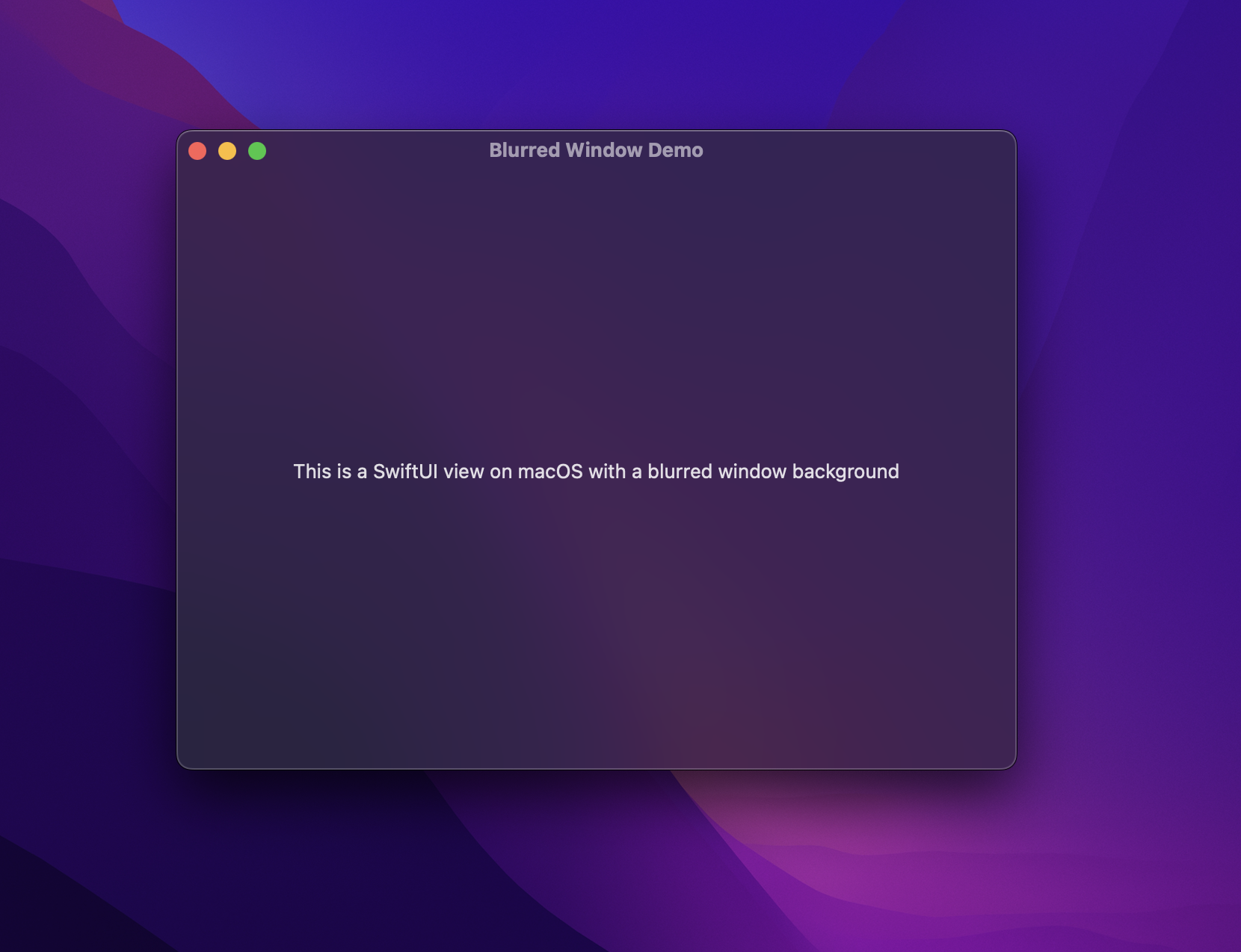
If you want more configuration for the VisualEffectView
, you can add additional properties to the representable struct to configure the underlying NSVisualEffectView
.
The full code and demo app is available here - https://github.com/zachwaugh/swiftui-examples.